整个项目源代码可以到我的github上下载。表单验证主要使用JQuery实现,IDE为IDEA。
导入项目
新建一个模块
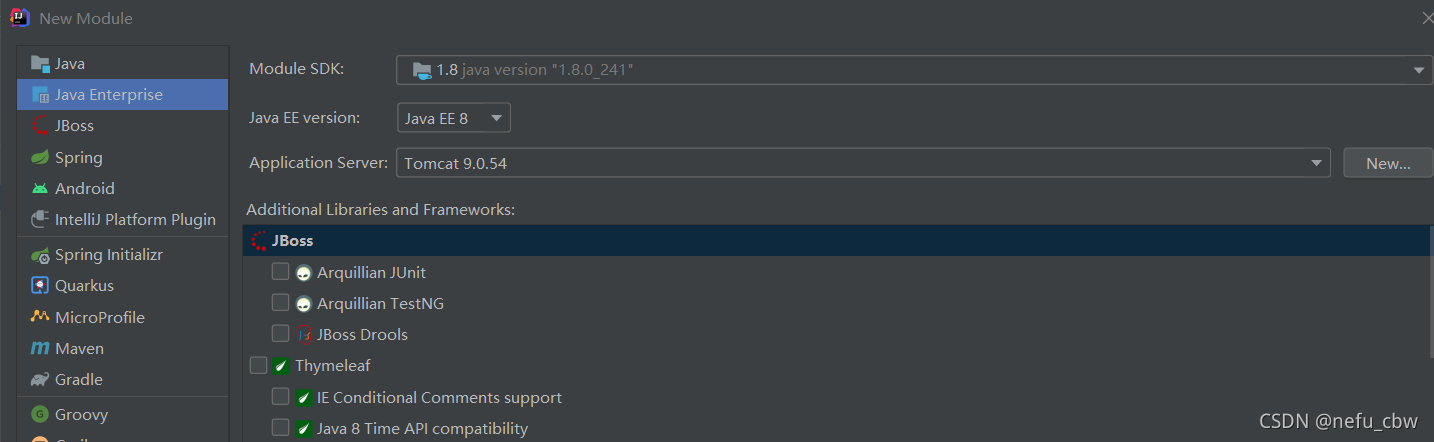
把原有的文件导入,原有文件链接 提取码:nefu
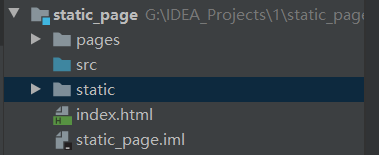
接下来我们要修改login.html以及regist.html
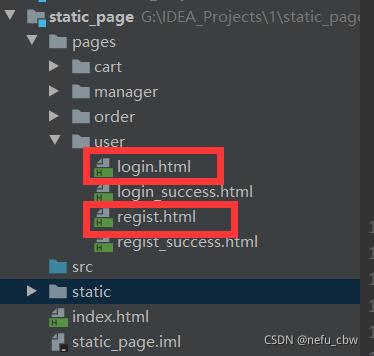
把 jquery.js
放入static/script
文件夹下
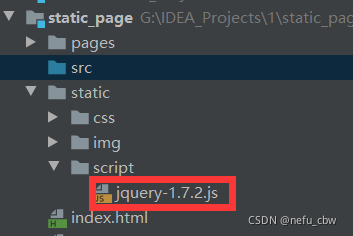
regist部分
我们要验证表单内容,主要有以下几个部分
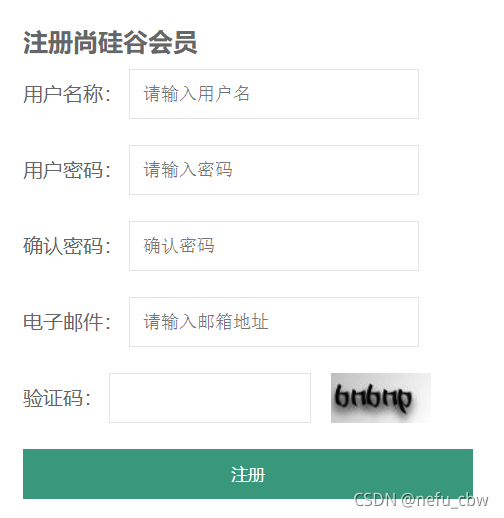
- 验证用户名:必须由字母,数字下划线组成,并且长度为5到12位
- 验证密码:必须由字母,数字下划线组成,并且长度为5到12位
- 验证确认密码:和密码相同
- 邮箱验证;xxxxx@xxx.com
- 验证码:现在只需要验证用户已输入
具体大致流程如下:
$(#id).val()
获得表单项的值
-
/ /
创建正则项表达式
- 使用
test
方法测试
$("span.errorMsg").text("提示信息")
提示用户
以用户名为例,具体代码如下
1 2 3 4 5 6 7 8 9 10 11
| var usernameText = $('#username').val(); var usernamePatt = /^\w{5,12}$/; if(!usernamePatt.test(usernameText)) { $("span.errorMsg").text("用户名不合法!"); return false; }
|
需要注意的是,全部验证完之后,不应该出现错误信息,所以使用 $("span.errorMsg").text("")
将其清空。
regist.html 全部代码如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140
| <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>尚硅谷会员注册页面</title> <link type="text/css" rel="stylesheet" href="../../static/css/style.css" > <script type="text/javascript" src="../../static/script/jquery-1.7.2.js"></script> <script type="text/javascript"> $(function () { $("#sub_btn").click(function () {
var usernameText = $('#username').val(); var usernamePatt = /^\w{5,12}$/; if(!usernamePatt.test(usernameText)) { $("span.errorMsg").text("用户名不合法!"); return false; }
var passwordText = $('#password').val(); var passwordPatt = /^\w{5,12}$/; if(!passwordPatt.test(passwordText)) { $("span.errorMsg").text("密码不合法!"); return false; }
var repwdText = $("#repwd").val(); if(repwdText != passwordText){ $("span.errorMsg").text("确认密码和密码不一致!"); return false; }
var emailText = $("#email").val(); var emailPatt = /^[a-z\d]+(\.[a-z\d]+)*@([\da-z](-[\da-z])?)+(\.{1,2}[a-z]+)+$/ if(!emailPatt.test(emailText)) { $("span.errorMsg").text("邮箱不合法!"); return false; }
var codeText = $("#code").val(); codeText = $.trim(codeText); if (codeText == null || codeText == ""){ $("span.errorMsg").text("验证码不能为空!"); return false; }
$("span.errorMsg").text(""); }); }); </script> <style type="text/css"> .login_form{ height:420px; margin-top: 25px; } </style> </head> <body> <div id="login_header"> <img class="logo_img" alt="" src="../../static/img/logo.gif" > </div> <div class="login_banner"> <div id="l_content"> <span class="login_word">欢迎注册</span> </div> <div id="content"> <div class="login_form"> <div class="login_box"> <div class="tit"> <h1>注册尚硅谷会员</h1> <span class="errorMsg"></span> </div> <div class="form"> <form action="regist_success.html"> <label>用户名称:</label> <input class="itxt" type="text" placeholder="请输入用户名" autocomplete="off" tabindex="1" name="username" id="username" /> <br /> <br /> <label>用户密码:</label> <input class="itxt" type="password" placeholder="请输入密码" autocomplete="off" tabindex="1" name="password" id="password" /> <br /> <br /> <label>确认密码:</label> <input class="itxt" type="password" placeholder="确认密码" autocomplete="off" tabindex="1" name="repwd" id="repwd" /> <br /> <br /> <label>电子邮件:</label> <input class="itxt" type="text" placeholder="请输入邮箱地址" autocomplete="off" tabindex="1" name="email" id="email" /> <br /> <br /> <label>验证码:</label> <input class="itxt" type="text" style="width: 150px;" id="code"/> <img alt="" src="../../static/img/code.bmp" style="float: right; margin-right: 40px"> <br /> <br /> <input type="submit" value="注册" id="sub_btn" /> </form> </div> </div> </div> </div> </div> <div id="bottom"> <span> 尚硅谷书城.Copyright ©2015 </span> </div> </body> </html>
|
演示结果
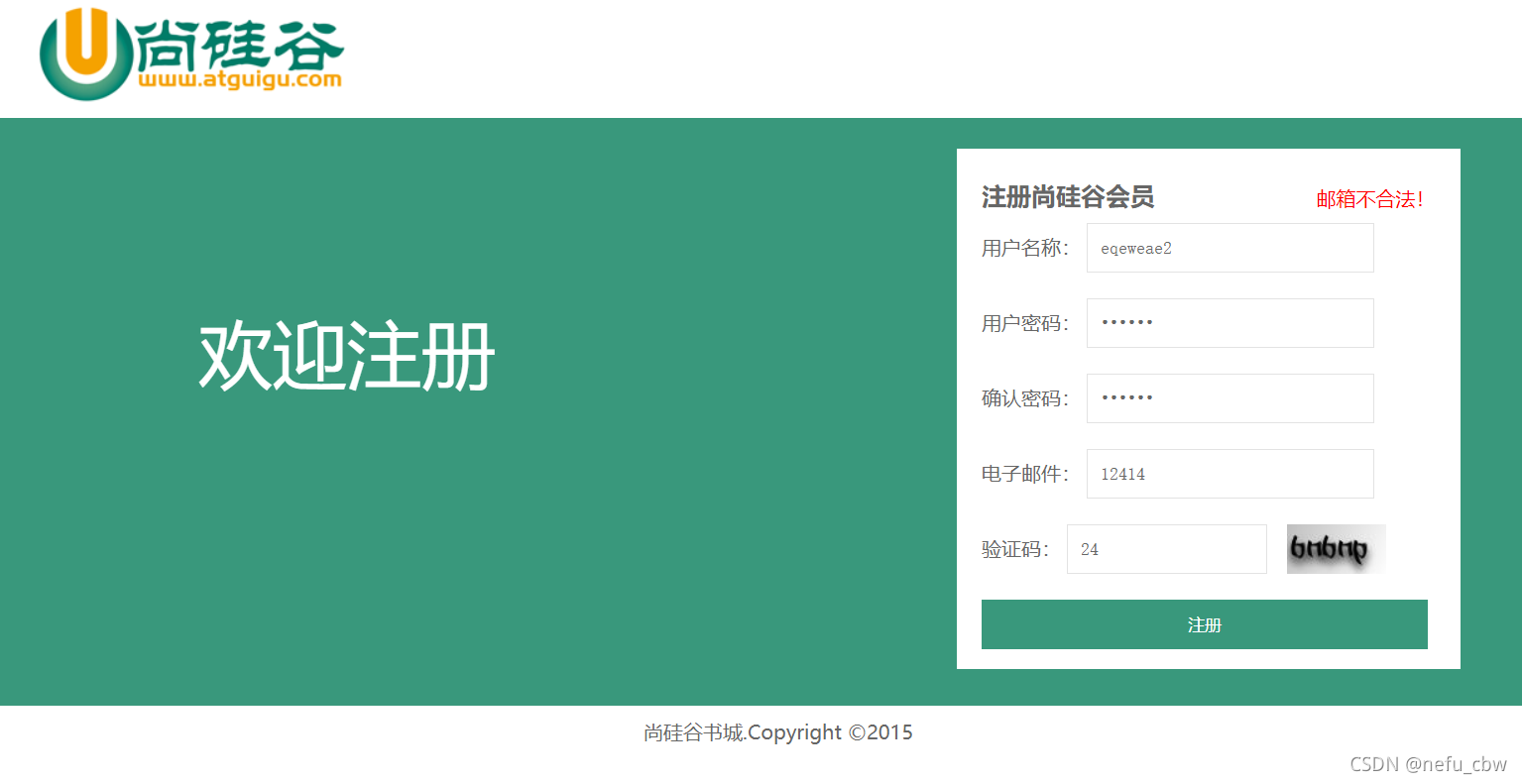
login部分
login部分也是一样的思路,因为表单标签没有提供 id
,我们要为其添加一个id
标签,即修改原始代码如下
1 2
| <input id="username" class="itxt" type="text" placeholder="请输入用户名" autocomplete="off" tabindex="1" name="username" /> <input id="password" class="itxt" type="password" placeholder="请输入密码" autocomplete="off" tabindex="1" name="password" />
|
后面就和注册一样的思路,利用 JQuery
验证表单即可。
login.html 全部代码如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89
| <!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>尚硅谷会员登录页面</title> <link type="text/css" rel="stylesheet" href="../../static/css/style.css" > <script type="text/javascript" src="../../static/script/jquery-1.7.2.js"></script> <script type="text/javascript"> $(function () { $("#sub_btn").click(function () {
var usernameText = $("#username").val(); var usernamePatt = /^\w{5,12}$/; if(!usernamePatt.test(usernameText)) { $("span.errorMsg").text("用户名不合法!"); return false; }
var passwordText = $("#password").val(); var passwordPatt = /^\w{5,12}$/; if(!passwordPatt.test(passwordText)) { $("span.errorMsg").text("密码不合法!"); return false; }
$("span.errorMsg").text(""); }); }); </script> </head> <body> <div id="login_header"> <img class="logo_img" alt="" src="../../static/img/logo.gif" > </div> <div class="login_banner"> <div id="l_content"> <span class="login_word">欢迎登录</span> </div> <div id="content"> <div class="login_form"> <div class="login_box"> <div class="tit"> <h1>尚硅谷会员</h1> <a href="regist.html">立即注册</a> </div> <div class="msg_cont"> <b></b> <span class="errorMsg">请输入用户名和密码</span> </div> <div class="form"> <form action="login_success.html"> <label>用户名称:</label> <input id="username" class="itxt" type="text" placeholder="请输入用户名" autocomplete="off" tabindex="1" name="username" /> <br /> <br /> <label>用户密码:</label> <input id="password" class="itxt" type="password" placeholder="请输入密码" autocomplete="off" tabindex="1" name="password" /> <br /> <br /> <input type="submit" value="登录" id="sub_btn" /> </form> </div> </div> </div> </div> </div> <div id="bottom"> <span> 尚硅谷书城.Copyright ©2015 </span> </div> </body> </html>
|
演示结果
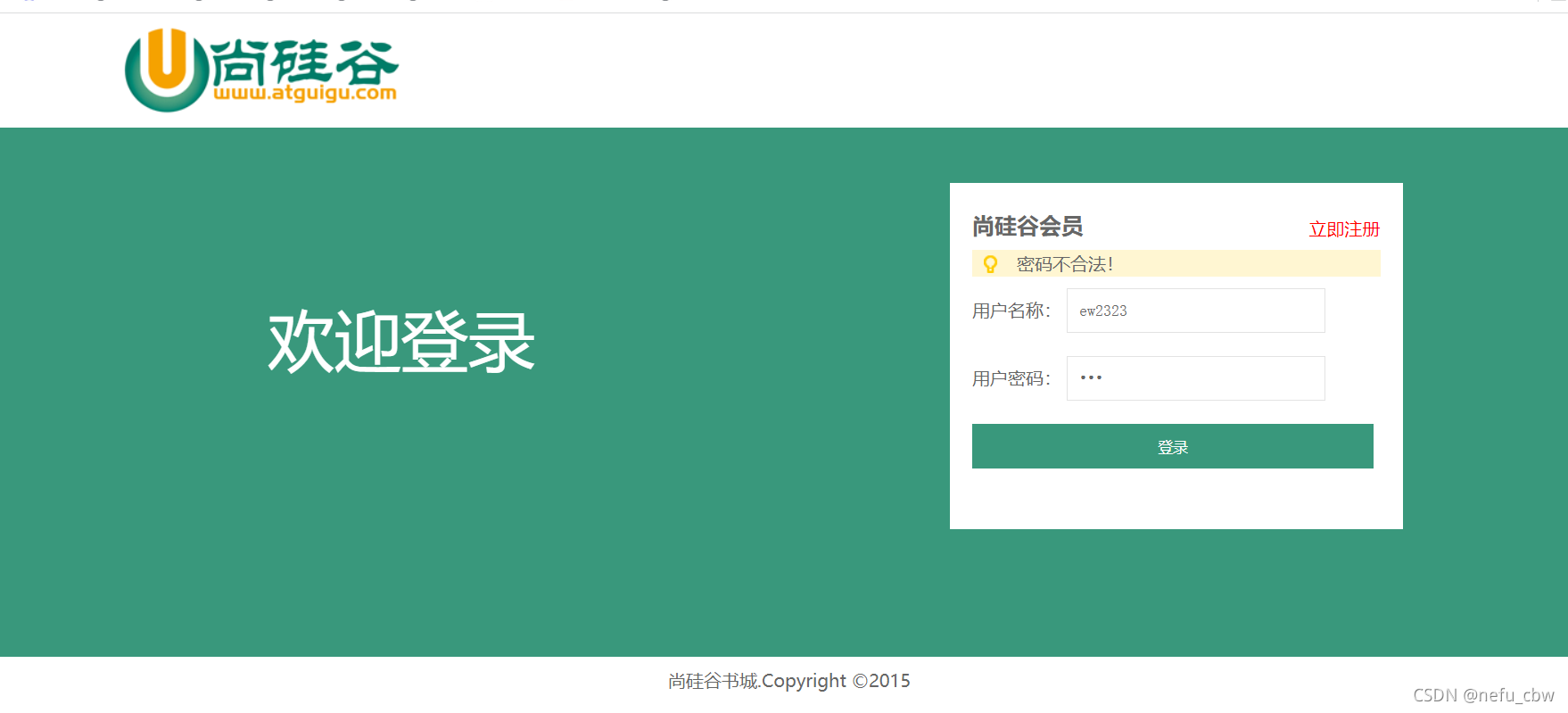